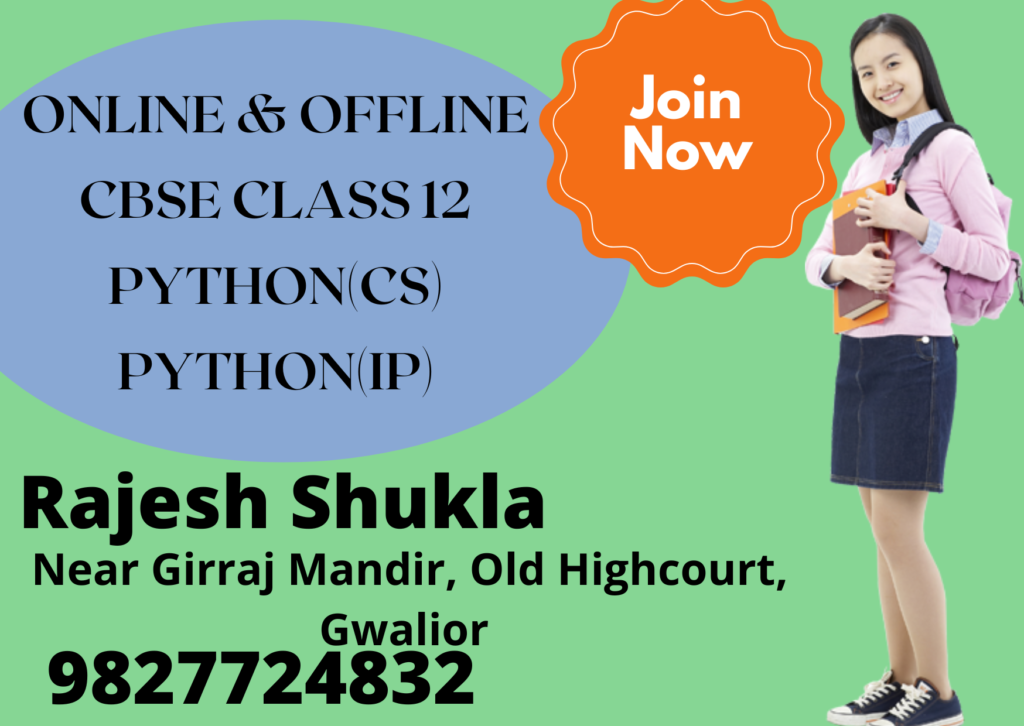
File reading: Word by word
Question:5
write a (python script) function named count_words() to read the contents of the file “abc.txt” word by word and display the contents. and also display total number of words not starting with “a” or “A” in the file.
Sol:
def count_words(): w=0 with open("abc.txt") as f: for line in f: for word in line.split(): if not(word[0]=="a" or word[0]=="A"): print(word) w=w+1 print("total words not starting with 'a' are ",w) function calling count_words()
Question:6
write a (python script) function named count_words() to read the contents of the file “abc.txt” word by word and display the contents. and also display total number of words ending with “t” or “T” in the file. and also display length of each word.
Sol:
def count_words(): w=0 with open("abc.txt") as f: for line in f: for word in line.split(): i=len(word) if(word[i-1]=="T" or word[i-1]=="t"): print(word," ",len(word)) w=w+1 print("total words ending with 't' are ",w) function calling count_words()
Question:7
write a (python script) function named count_words() to read the contents of the file “abc.txt” word by word and display the contents. and also display total number of words ending with vowels and also display length of each word.
Sol:
def count_words(): w=0 vowels='aeiouAEIOU' with open("abc.txt") as f: for line in f: for word in line.split(): i=len(word) if(word[i-1]in vowels): print(word," ",len(word)) w=w+1 print("total words ending with vowels are ",w) function calling count_words()
Question:8
write a (python script) function named count_words() to read the contents of the file “abc.txt” word by word. Check and print total “the” present in the file.
Sol:
def count_words(): w=0 with open("abc1.txt") as f: for line in f: for word in line.split(): n=word.lower() if(n=="the"): w=w+1 print("total words 'the' present: ",w) function calling count_words()
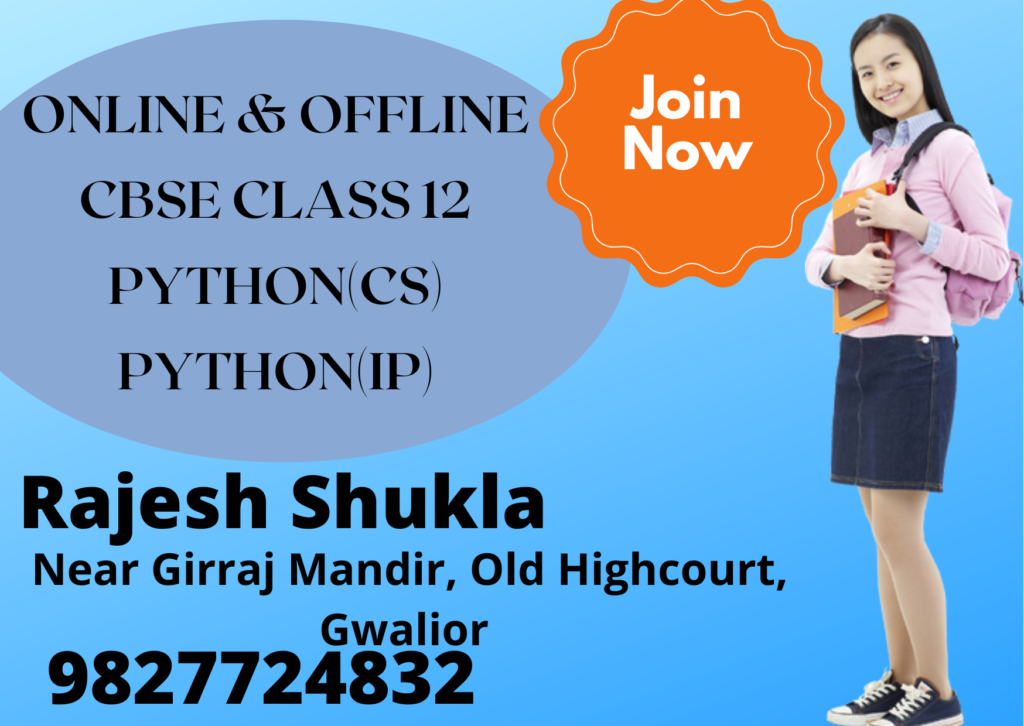
Question:9
write a (python script) function named count_words() to read the contents of the file “abc.txt” word by word. Check and print total “this” present in the file.
Sol:
def count_words(): w=0 with open("abc1.txt") as f: for line in f: for word in line.split(): n=word.lower() if(n=="this"): w=w+1 print("total words 'this' present: ",w) function calling count_words()