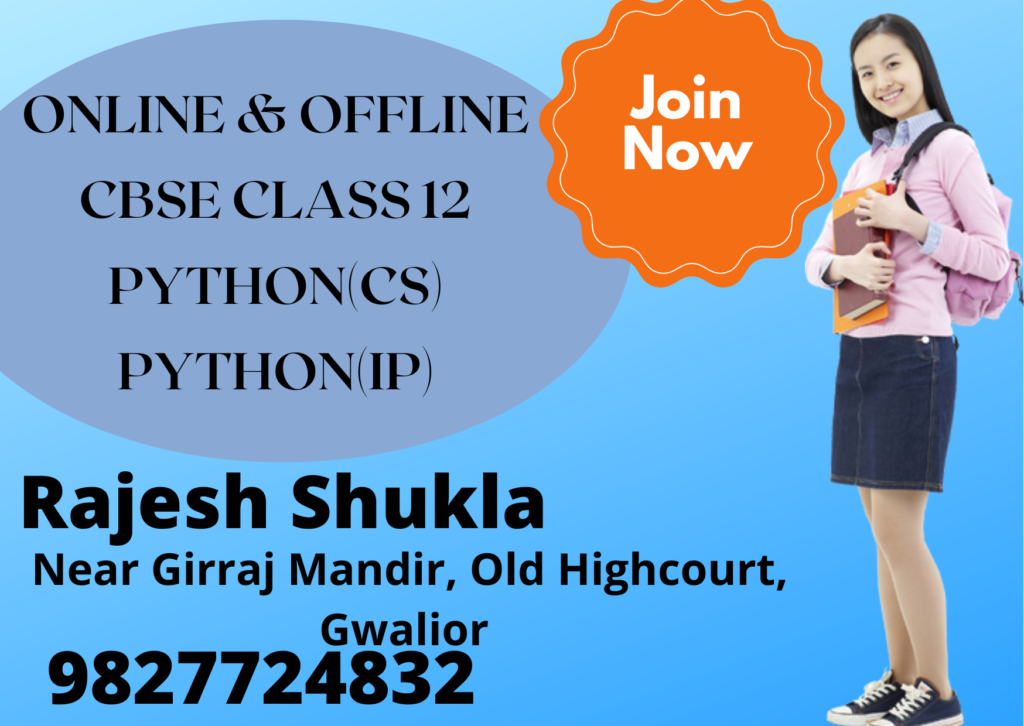
File Reading: Line by Line
Question:1
Python Program to read a file “story.txt” line by line and display the contents
Sol:
Method:1
filepath = 'story.txt' with open(filepath) as fp: line = fp.readline() cnt = 1 while line: print("Line {}: {}".format(cnt, line.strip())) line = fp.readline() cnt += 1
Method:2
filepath = 'story.txt' with open(filepath) as fp: line = fp.readline() cnt = 1 while line: print(cnt," ",line,end='') line = fp.readline() cnt += 1
Question: 2
Python Program to read a file “story.txt” line by line and display the contents. Further count and print total lines in the file
Sol:
Method:1
filepath = 'story.txt' with open(filepath) as fp: line = fp.readline() cnt = 0 while line: cnt += 1 print("Line {}: {}".format(cnt, line.strip())) line = fp.readline() print("Total lines in file ",cnt)
Method:2
filepath = 'story.txt' with open(filepath) as fp: line = fp.readline() cnt = 0 while line: cnt += 1 print(cnt," ",line,end='') line = fp.readline() print("Total lines in file ",cnt)
Question: 3
Python Program to read a file “story.txt” line by line and display the contents. count and print total lines starting with “A” or “a” in the file
#Note:
Using the above program we can display line starting with any character.
Sol:
Method:1
filepath = 'story.txt' with open(filepath) as fp: line = fp.readline() cnt = 1 while line: if(line[0]=='a' or line[0]=='A'): #print(line) print("Line {}: {}".format(cnt, line.strip())) cnt=cnt+1 line = fp.readline()
Method:2
filepath = 'story.txt' with open(filepath) as fp: line = fp.readline() cnt = 1 while line: if(line[0]=='a' or line[0]=='A'): #print(line) print(cnt," ",line,end='') cnt=cnt+1 line = fp.readline()
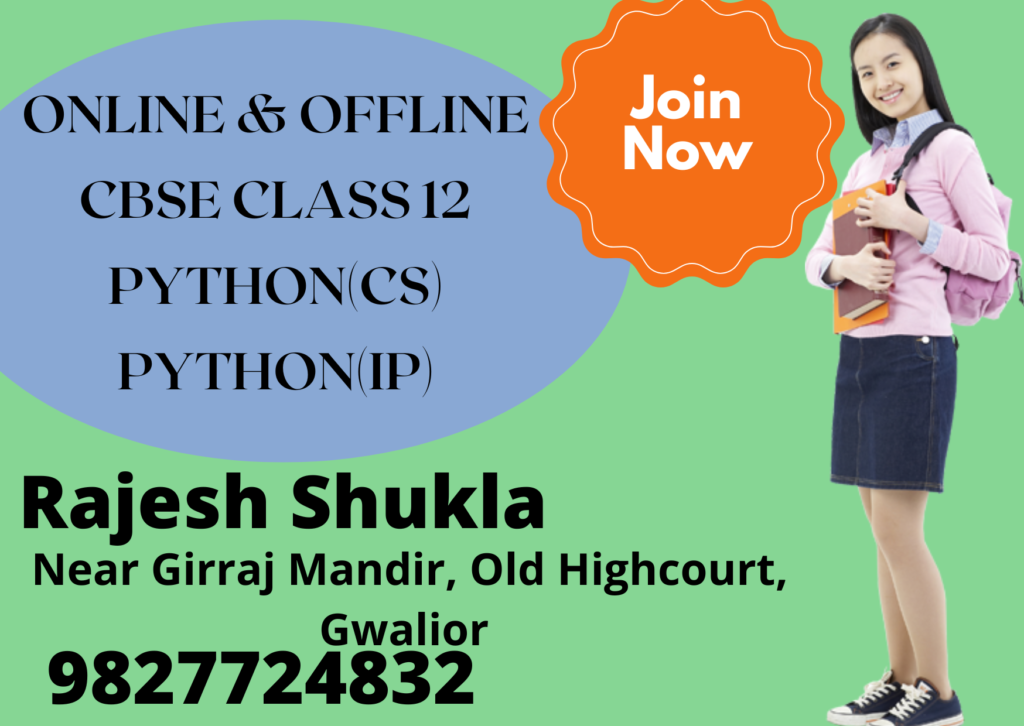
Question: 4
Python Program to read a file “story.txt” line by line and display the contents. count and print total lines starting with vowels (A,a,E,e,I,i,O,o,U,u) in the file
#Note:
Using the above program we can display line starting with any character.
Sol:
Method:1
filepath = 'story.txt' vowels="AEIOUaeiou" with open(filepath) as fp: line = fp.readline() cnt = 1 while line: if(line[0] in vowels): #print(line) print("Line {}: {}".format(cnt, line.strip())) cnt=cnt+1 line = fp.readline()
Method:2
filepath = 'story.txt' vowels="AEIOUaeiou" with open(filepath) as fp: line = fp.readline() cnt = 1 while line: if(line[0] in vowels): #print(line) print(cnt," ",line,end='') cnt=cnt+1 line = fp.readline()