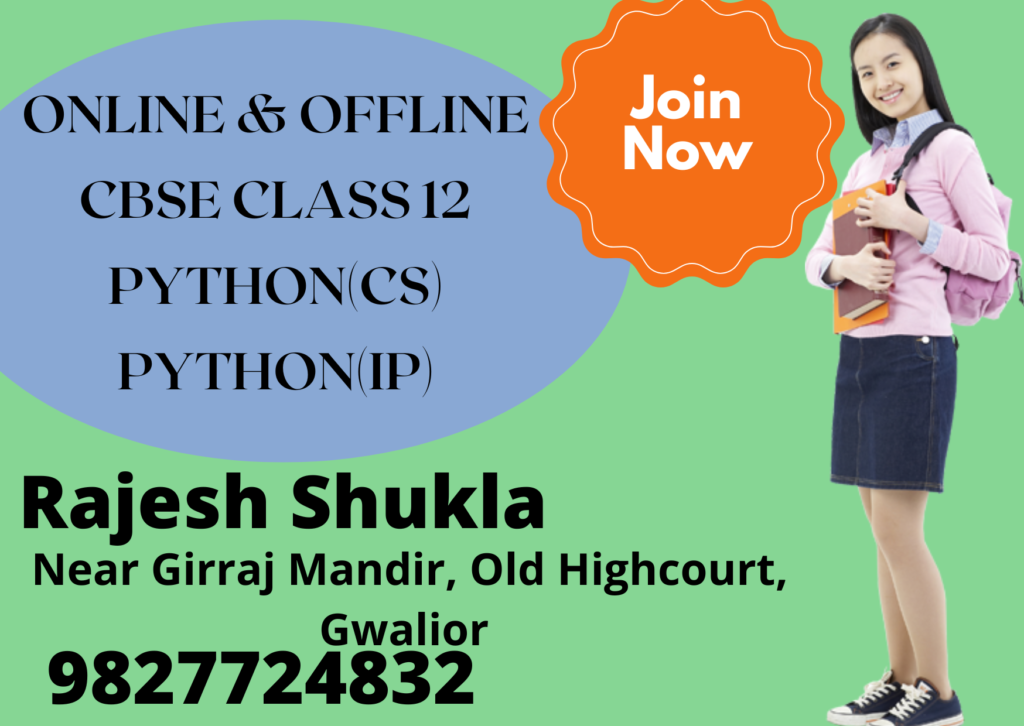
with statement
Apart from using open() or file() function for the creation of a file, “with statement” can also be used for the same purpose. We can use this statement to group file operations within a block. Using “with” ensures that all the resources allocated to the file object get deallocated automatically once we stop using the file. In the case of exceptions also, we are not required to close the file explicitly using “with” statement.
syntax:
with open(filename) as fileobject:
statements
statements
Example of “with” statement:
Python program to write contents into a file named “abc.txt”
#to write contents in the file with open("abc.txt","w") as f: f.write("hello how are you\n") f.write("welcome to python file handling\n") f.write("end of the file\n") #close the file f.close()
Now to read the contents of the above created file and display them.
#to read the contents of the file f=open("abc.txt","r") data=f.readlines() for i in data: print(i,end='') #close the file f.close()
Output:
hello how are you welcome to python file handling end of the file >>>
Appending data to a file
Append means “to add to”; so if we want to add more data to a file that already has some data in it, we have to open the file in append mode. In such a case, we use access mode “a” which means “open for writing, and if it exists, then append data to the end of the file”.
In Python, we can use the “a” mode to open an output file in append mode. this means that:
* if the file already exists, it will not be erased. if the file does not exist, it will be created.
* When data is written to the file, it will be written at the end of the file’s current contents.
Example of append mode:
Python program to write contents into a file named “abc.txt”
#to write contents in the file with open("abc.txt","w") as f: f.write("hello how are you\n") f.write("welcome to python file handling\n") f.write("end of the file\n") #close the file f.close()
#to write contents in the file in append mode with open("abc.txt","a") as f: f.write("This is new data line 1\n") f.write("This is new data line 2\n") f.write("end of the file\n") #close the file f.close()
Now to read the contents of the above created file and display them.
#to read the contents of the file f=open("abc.txt","r") data=f.readlines() for i in data: print(i,end='') #close the file f.close()
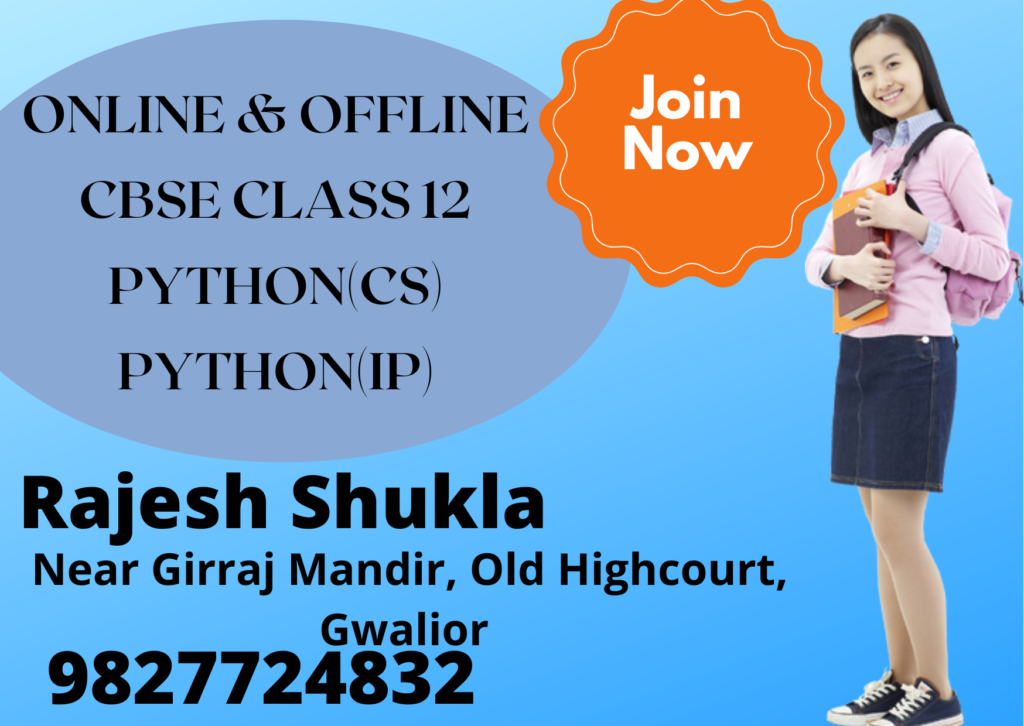
Output:
hello how are you welcome to python file handling end of the file This is new data line 1 This is new data line 2 end of the file >>>