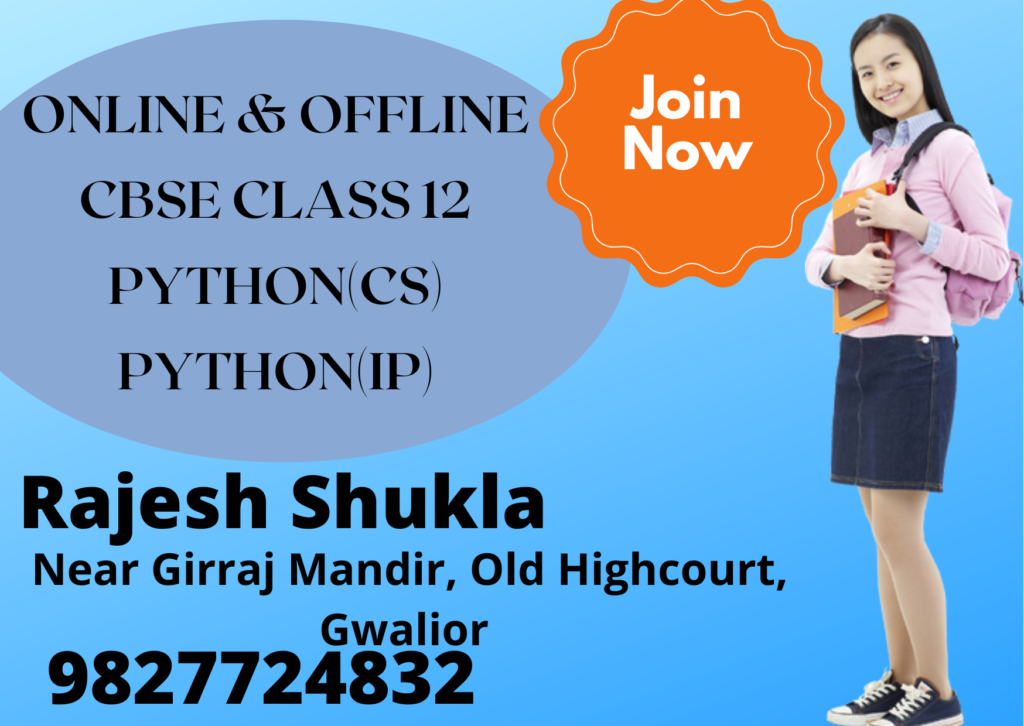
File reading: Character By Character
Question:1
Write a python script to read the contents of a file character by character and display them?(Method:1)
Sol:
def file_read(): f=open("abc.txt") while True: c=f.read(1) if not c: #print("end of file") break print(c,end='') #function calling file_read()
Question:2
Write a python script to read the contents of a file character by character and display them? (Method:2)
Sol:
def file_read1(): with open("abc.txt") as f: while True: c=f.read(1) if not c: #print("end of file") break print(c,end='') #function calling file_read1()
Question:3
write a (python script) function named count_len() to read the contents of the file “abc.txt” and print its length.
Sol:
def count_len(): le=0 with open("abc.txt") as f: while True: c=f.read(1) if not c: break print(c,end='') le=le+1 print("length of file ",le) #function calling count_len()
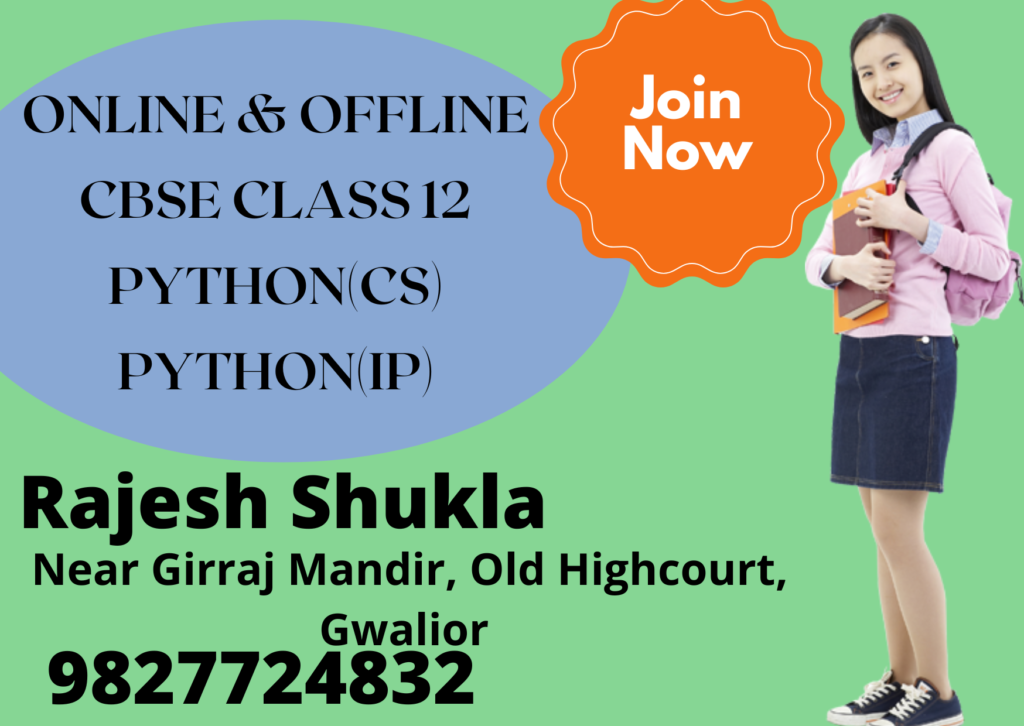
Question:4
write a (python script) function named count_lower() to read the contents of the file “story.txt”. Further count and print total lower case alphabets in the file
Sol:
def count_lower(): lo=0 with open("story.txt") as f: while True: c=f.read(1) if not c: break print(c,end='') if(c>='a' and c<='z'): lo=lo+1 print("total lower case alphabets ",lo) function calling count_lower()
Question:5
write a (python script) function named count_upper() to read the contents of the file “story.txt”. Further count and print total upper case alphabets in the file.
Sol:
def count_upper(): lo=0 with open("story.txt") as f: while True: c=f.read(1) if not c: break print(c,end='') if(c>='A' and c<='Z'): lo=lo+1 print("total upper case alphabets ",lo) function calling count_upper()