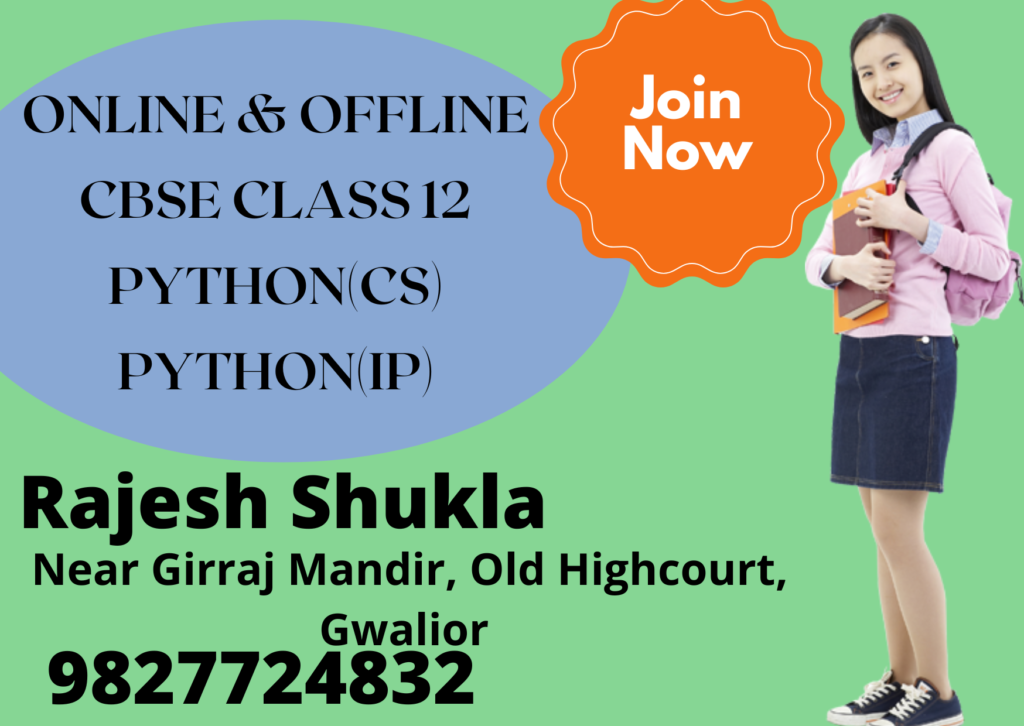
Functions with arguments and not returning any value
In this we pass arguments to the function , but function does not return any value.
Example:
Question:1
Python script to calculate and print sum of two numbers
Sol:
#function definition def sum(p,q): s=p+q print("Sum = ",s) #function calling a=int(input("Enter 1st no ")) b=int(input("Enter 2nd no ")) sum(a,b)
Output:
Enter 1st no 10
Enter 2nd no 20
Sum = 30
>>>
Question:2
Python script to calculate and print square of a number
Sol:
#function definition def square(n): s=n*n print("Square = ",s) #function calling a=int(input("Enter 1st no ")) square(a)
Output:
Enter 1st no 2
Square = 4
>>>
Question:3
Python script to calculate and print cube of a number
Sol:
#function definition def cube(n): s=n*n*n print("Cube = ",s) #function calling a=int(input("Enter 1st no ")) cube(a)
Output:
Enter 1st no 5
Cube = 125
>>>
Question:4
Python script to calculate and print square and cube of a number(Multiple functions)
Sol:
#function definition def square(n): s=n*n print("square = ",s) def cube(n): s=n*n*n print("Cube = ",s) #function calling a=int(input("Enter 1st no ")) square(a) cube(a)
Output:
Enter 1st no 5
square = 25
Cube = 125
>>>
Question:5
Python script to calculate and print sum , product difference and average of two numbers
Sol:
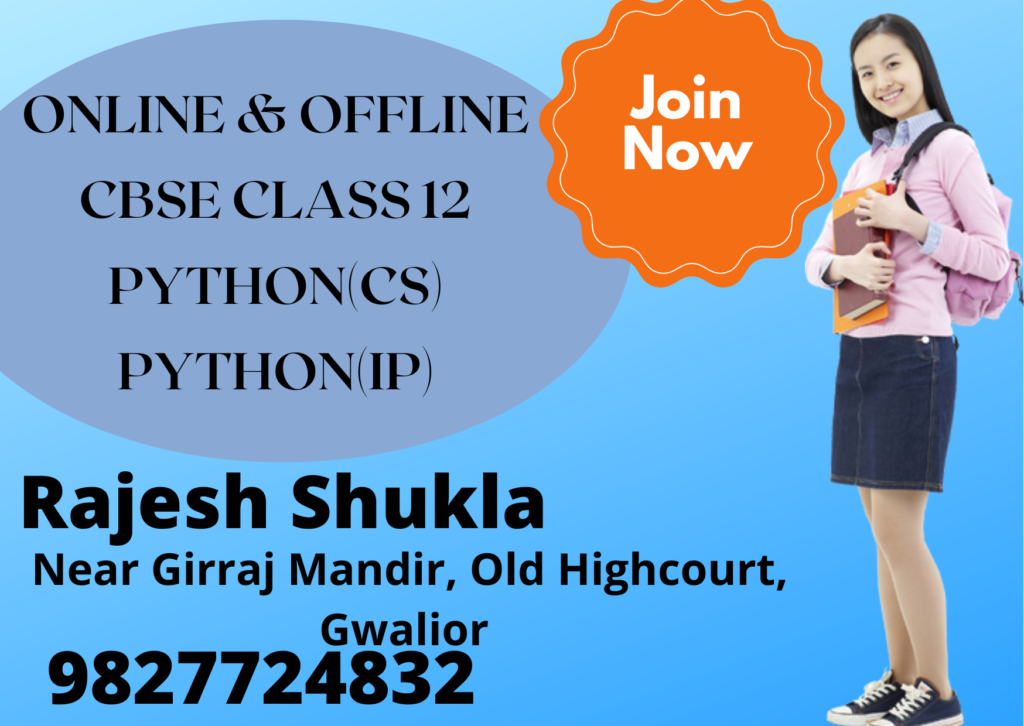
#function definition def calculate(n1,n2): s=n1+n2 p=n1*n2 if(n1>n2): d=n1-n2 else: d=n2-n1 av=(n1+n2)/2 print("sum = ",s) print("Prod = ",p) print("Diff = ",d) print("average = ",av) #function calling a=int(input("Enter 1st no ")) b=int(input("Enter 2nd no ")) calculate(a,b)
Output:
Enter 1st no 10
Enter 2nd no 20
sum = 30
Prod = 200
Diff = 10
average = 15.0
>>>
Question:6
Python script to calculate and print sum , product difference and average of two numbers(Multiple functions)
Sol:
#function definition def sum(n1,n2): s=n1+n2 print("sum = ",s) def prod(n1,n2): p=n1*n2 print("Prod = ",p) def diff(n1,n2): if(n1>n2): d=n1-n2 else: d=n2-n1 print("Diff = ",d) def average(n1,n2): av=(n1+n2)/2 print("average = ",av) #function calling a=int(input("Enter 1st no ")) b=int(input("Enter 2nd no ")) sum(a,b) prod(a,b) diff(a,b) average(a,b)
Output:
Enter 1st no 10
Enter 2nd no 20
sum = 30
Prod = 200
Diff = 10
average = 15.0
>>>