By: Archana Shukla and Rajesh Shukla
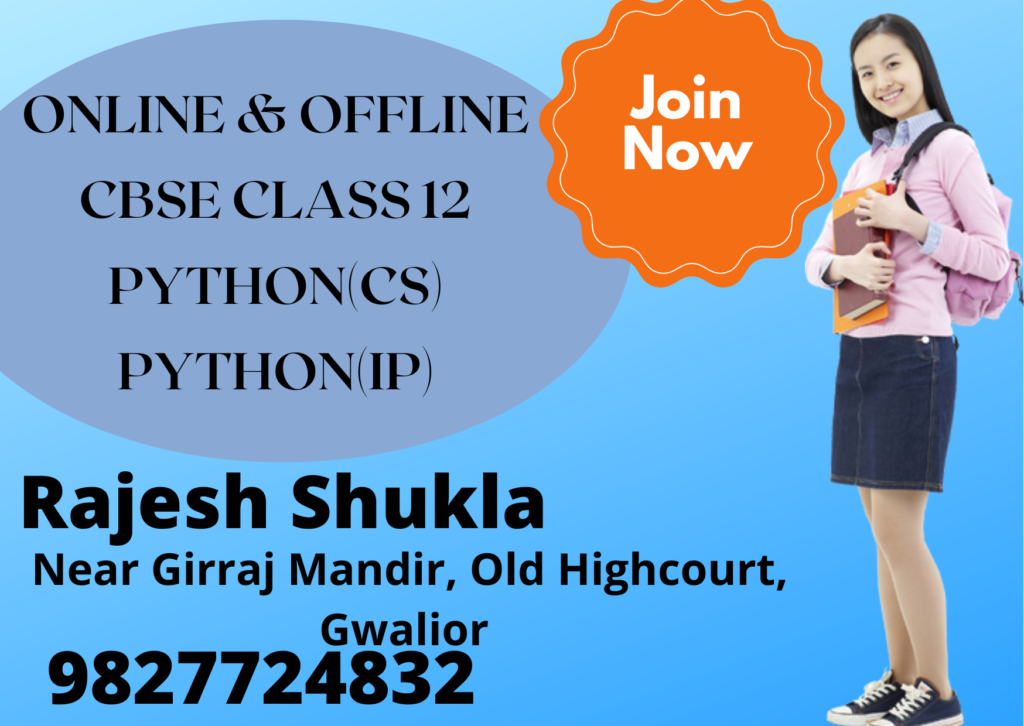
Functions without arguments and not returning any values
In this, we do not pass any arguments to the function and the function does not return any value.
Examples:
Question:1
Write a python script using functions to calculate and print square of a number
Sol:
#function definition def square(): a=int(input("Enter any no ")) b=a*a print("Square = ",b) #function calling square()
Output:
Enter any no 5
Square = 25
>>>
Question:2
Write a python script using function to calculate and print cube of a number
Sol:
#function definition def cube(): a=int(input("Enter any no ")) b=a*a*a print("Cube = ",b) #function calling cube()
Output:
Enter any no 5
Cube = 125
>>>
Question:3
Write a python script using function to take input for two numbers calculate and print their sum, product and difference.
Sol:
Method:1 Using Single Function
#function definition def calculate(): a=int(input("Enter 1st no ")) b=int(input("Enter 2nd no ")) s=a+b p=a*b; if(a>b): d=a-b else: d=b-a; print("Sum = ",s) print("Prod = ",p) print("Diff = ",d) #function calling calculate()
Output:
Enter 1st no 10
Enter 2nd no 20
Sum = 30
Prod = 200
Diff = 10
>>>
Method:2 (Using Multiple functions)
#function definition def sum1(): a=int(input("Enter 1st no ")) b=int(input("Enter 2nd no ")) s=a+b print("Sum = ",s) def prod(): a=int(input("Enter 1st no ")) b=int(input("Enter 2nd no ")) p=a*b print("prod = ",p) def diff(): a=int(input("Enter 1st no ")) b=int(input("Enter 2nd no ")) if(a>b): d=a-b else: d=b-a print("diff = ",d) def avg(): a=int(input("Enter 1st no ")) b=int(input("Enter 2nd no ")) av=(a+b)/2 print("avg = ",av) #function calling sum1() prod() diff() avg()
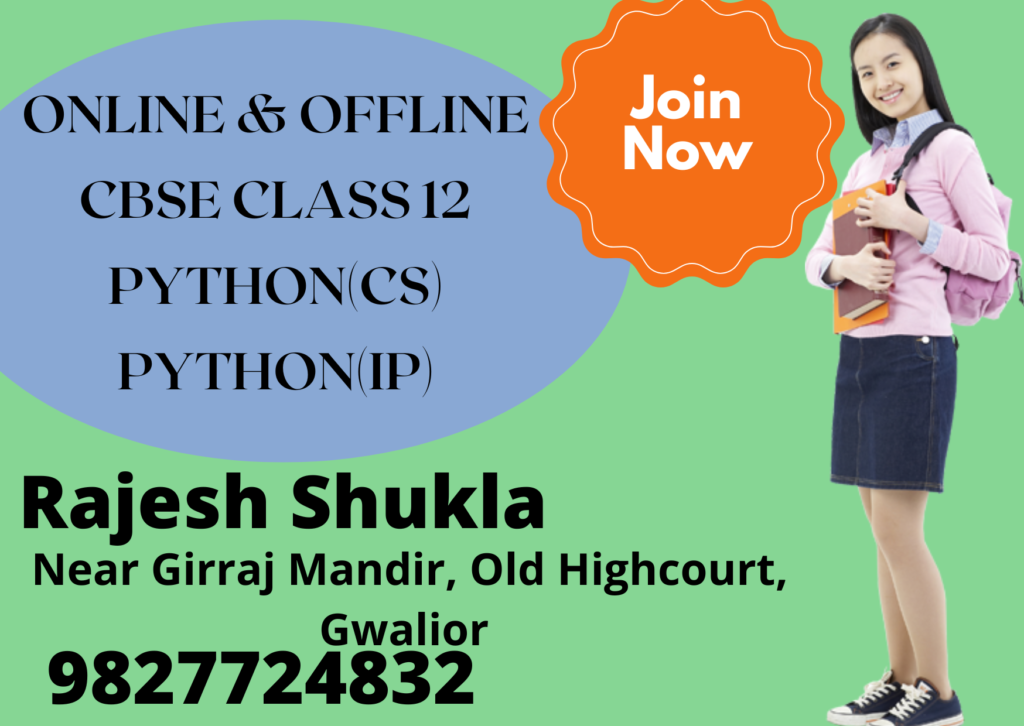
Output:
Enter 1st no 10
Enter 2nd no 20
Sum = 30
Enter 1st no 2
Enter 2nd no 3
prod = 6
Enter 1st no 25
Enter 2nd no 6
diff = 19
Enter 1st no 2
Enter 2nd no 6
avg = 4.0
>>>
Question:4
Write a python using function to take input for 3 numbers check and print the maximum number.
Sol:
#function definition def largest(): a=int(input("Enter 1st no ")) b=int(input("Enter 2nd no ")) c=int(input("Enter 2nd no ")) if(a>b and a>c): m=a elif(b>c): m=b else: m=c print("Max no = ",m) #function calling largest()
Output:
Enter 1st no 25
Enter 2nd no 96
Enter 2nd no 3
Max no = 96
>>>
Question:5
Write a python script using function to take input for 3 numbers check and print the lowest number.
Sol:
#function definition def lowest(): a=int(input("Enter 1st no ")) b=int(input("Enter 2nd no ")) c=int(input("Enter 2nd no ")) if(a<b and a<c): m=a elif(b<c): m=b else: m=c print("Min no = ",m) #function calling lowest()
Output:
Enter 1st no 25
Enter 2nd no 963
Enter 2nd no 4
Min no = 4
>>>