By: Archana Shukla and Rajesh Shukla
A User-defined Python function is a group of related statements that helps us to perform a specific task.
- The use of functions improves the clarity and readability of the program.
- It helps to break our program into smaller and modular chunks.
- As our program grows larger and larger, functions make it more organized and manageable.
- The function makes them more efficient by reducing duplication of code.
- It breaks the complex tasks into more manageable parts.
Functions are also known as methods, modules, procedures, routines, sub-routines, or sub-program.
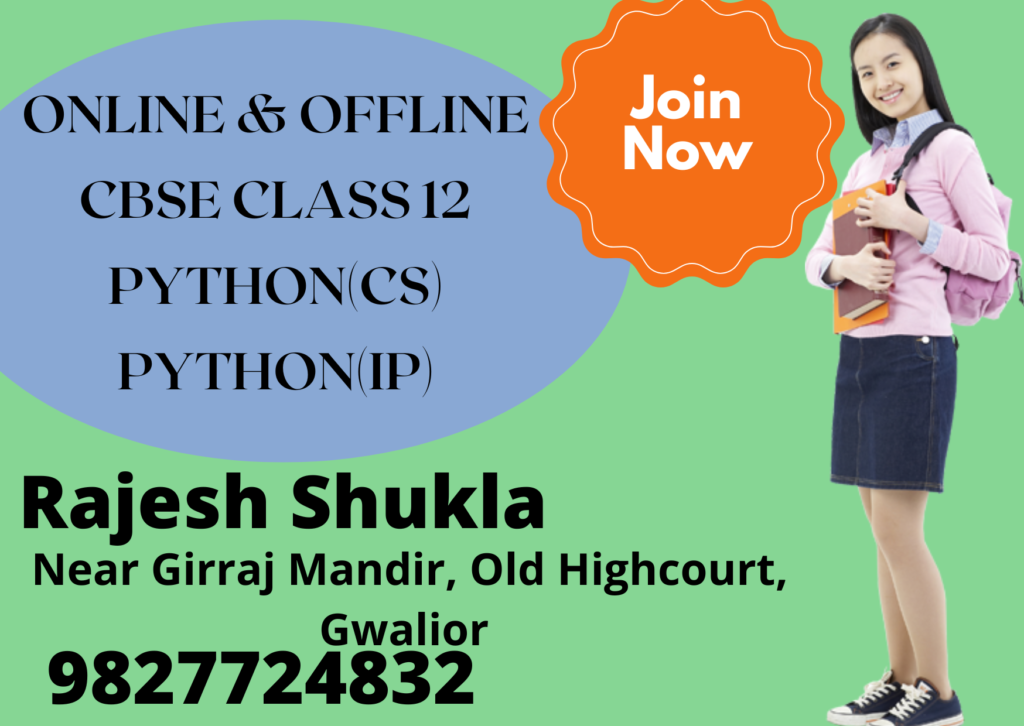
How to define a function
A user-defined python function is created using the keyword “def”.
Syntax of Function
#function definition def function_name(list_of_parameters): """ docstring """ statements statements statements return variable
A function definition in python consists of the following components.
def
- The keyword “def” marks the start of the function header.
function_name
- A function name helps us to identify the function.
- The function name should follow the rules of writing identifiers in Python.
List_of_parameters
- These help us to pass values to a function.
- These are optional.
colon (:)
- This marks the end of the function header.
docstring
- Optional documentation string (docstring) to describe the purpose/objective of the function.
- documentation is a good programming practice.
statements
- One or more valid python statements that make up the function body.
Statements must have the same indentation level.
return variable
- An optional return statement to return a value from the function.
Example:
def hello(): """ this is function named hello """ print("we are in hello function") def hi(): ''' this is function hi to test function ''' print("this is good function") #function calling print("to call a function ") hello() #calling function hello() hi() #calling function hi() print() #just to leave blank line print("printing doc string") print(hello.__doc__) #to display doc string of function hello print(hi.__doc__) #to display doc string of function hello
Output:
to call a function
we are in hello function
this is good functionprinting doc string
this is function named hello
this is function hi
to test function
>>>
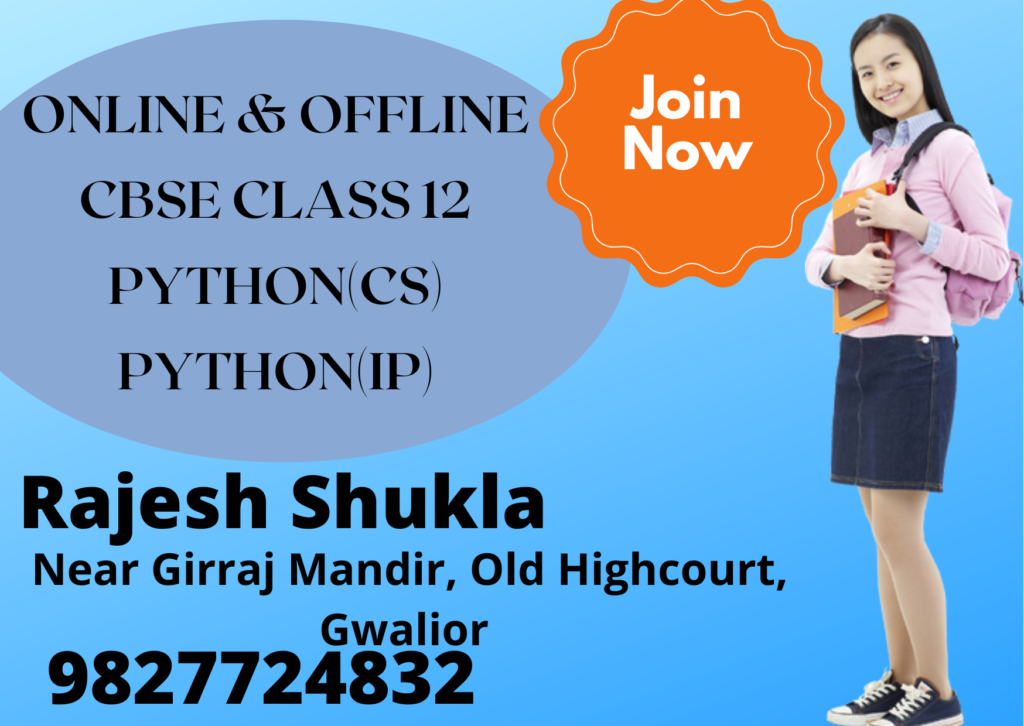
User-defined functions can further be divided into the following types:
* Functions without arguments
* Functions with arguments without returning any value
* function with arguments and returning value
* In Python a function can return multiple values(Very Important)